This HC-SR04 ultrasonic rangefinder has been enhanced with the LM35 temperature sensor.
From school you can recall that the speed of sound depends on the density of air, and the density of air depends on temperature.
We will calculate the temperature using this formula
temp2 = (temp / 1023.0) * 5.0 * 1000/10;
Where temp2 is the finished temperature value after the formula,
And where temp is the raw value from the sensor.
What we need:
- Ultrasonic Rangefinder HC-SR04
- Arduino
- Bread board
- Jumpers
- Thermistor LM35 (Temperature Sensor)
Keep in mind that HC-SR04 comes in two forms -
Here I have a good one and it stably measures distance.
They both measure from 4 cm to 400 cm.
Powered by 3.3 to 5 v
To begin with, we mock it all up on a breadboard.
Ultrasonic Sensor:
- GND - gnd
- Echo - 3 pins
- Trig - 2 pins
- Vcc - 5v
Thermistor LM35:
- 1 - 5v
- 2 - A0
- 3 - GND
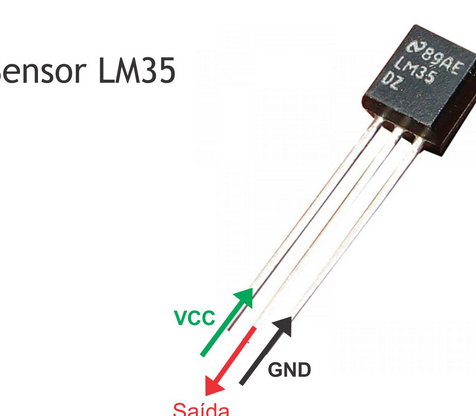
It should be like this:
Next we fill in the sketch.
Download library for HC-SR04 sensor -
Download sketch -
#include // connect the library to work with the ultrasonic sensor HC-SR04
iarduino_HC_SR04 hcsr (2,3); // denote contacts Trig and Echo
int temp = 0; // raw temperature
float temp2 = 0; // ready temperature value
void setup () {// run 1 time
Serial.begin (9600); // start the port monitor
pinMode (A0, INPUT); // denote contact A0 as input
}
void loop () {// repeats an infinite number of times
temp = analogRead (A0); // read the value of contact A0 and assign the variable temp to it
temp2 = (temp / 1023.0) * 5.0 * 1000/10; // calculate the raw value according to the formula to get the finished temperature value
Serial.println (hcsr.distance (temp2)); // write the ready range in the port monitor
delay (1000); // delay 1 second
}
And here is a sketch for an LCD display-
Download sketch -
#include // connect the library to work with the ultrasonic sensor HC-SR04
#include // connect the library to work with the display on the I2C bus
LiquidCrystal_I2C lcd (0x27, 16, 2); // denote (address, columns, lines)
iarduino_HC_SR04 hcsr (2,3); // denote contacts Trig and Echo
int temp = 0; // raw temperature
float temp2 = 0; // ready temperature value
void setup () {// run 1 time
lcd.init (); // initialize the display
lcd.backlight (); // turn on the display backlight
pinMode (A0, INPUT); // denote contact A0 as input
}
void loop () {// repeats an infinite number of times
temp = analogRead (A0); // read the value of contact A0 and assign the variable temp to it
temp2 = (temp / 1023.0) * 5.0 * 1000/10; // calculate the raw value according to the formula to get the finished temperature value
lcd.setCursor (2, 0); // put the cursor on 2 column and 0 row
lcd.print (temp2); // write the distance
lcd.setCursor (5,0); // place the cursor on the 5th column and 0th row
lcd.print ("cm"); // write cm
delay (1000); // delay 1 second
}
Write comments and questions, I will answer everything!